Alright, let me tell you about this “dim sum library menu” thing I cooked up. It was more of a “can I even do this?” kind of project than something I desperately needed, you know?
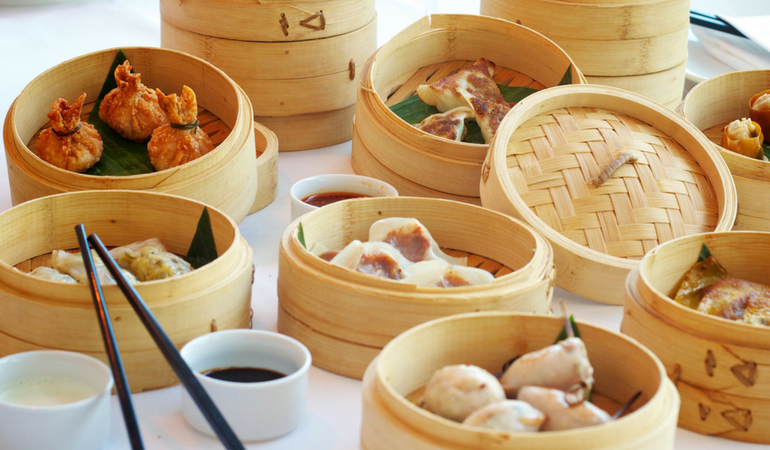
It all started when I was messing around with some data. I had this messy collection of dim sum names and descriptions, and I thought, “Wouldn’t it be cool if I could just, like, turn this into a menu-like structure in my code?” Not a real menu, mind you, just a way to organize and access the data easily. So, I Googled around a bit, found some stuff about Python dictionaries and lists, and thought, “Yeah, I can probably hack something together.”
First, I grabbed all the dim sum info. This was the painful part – copying and pasting from different websites, correcting typos, the whole shebang. I dumped everything into a text file. Then I had to figure out how to read this text file into my Python script. I remember wrestling with file encodings for a good hour before I realized I just needed to add “encoding=’utf-8′” when I opened the file. Rookie mistake, I know.
Next, I started thinking about how to structure the data. I decided to use a dictionary where each key was a category of dim sum (like “Steamed,” “Fried,” “Baked”) and each value was a list of dim sum items in that category. Each dim sum item would be another dictionary with “name” and “description” keys. Does that make sense?
The coding part was pretty straightforward, actually. I used a bunch of string splitting and list appending to parse the text file and build the dictionary. It looked something like this (simplified, of course):
dim_sum_menu = {}
with open('dim_sum_*', 'r', encoding='utf-8') as f:
for line in f:
category, name, description = *().split('') # Assuming data is separated by pipes
if category not in dim_sum_menu:
dim_sum_menu[category] = []
dim_sum_menu[category].append({'name': name, 'description': description})
Of course, there were tons of tweaks and error handling I had to add. Like, some lines in the text file were messed up, so I had to add some `try…except` blocks to skip them. And I wanted to make the menu look nice when I printed it out, so I added some formatting code.
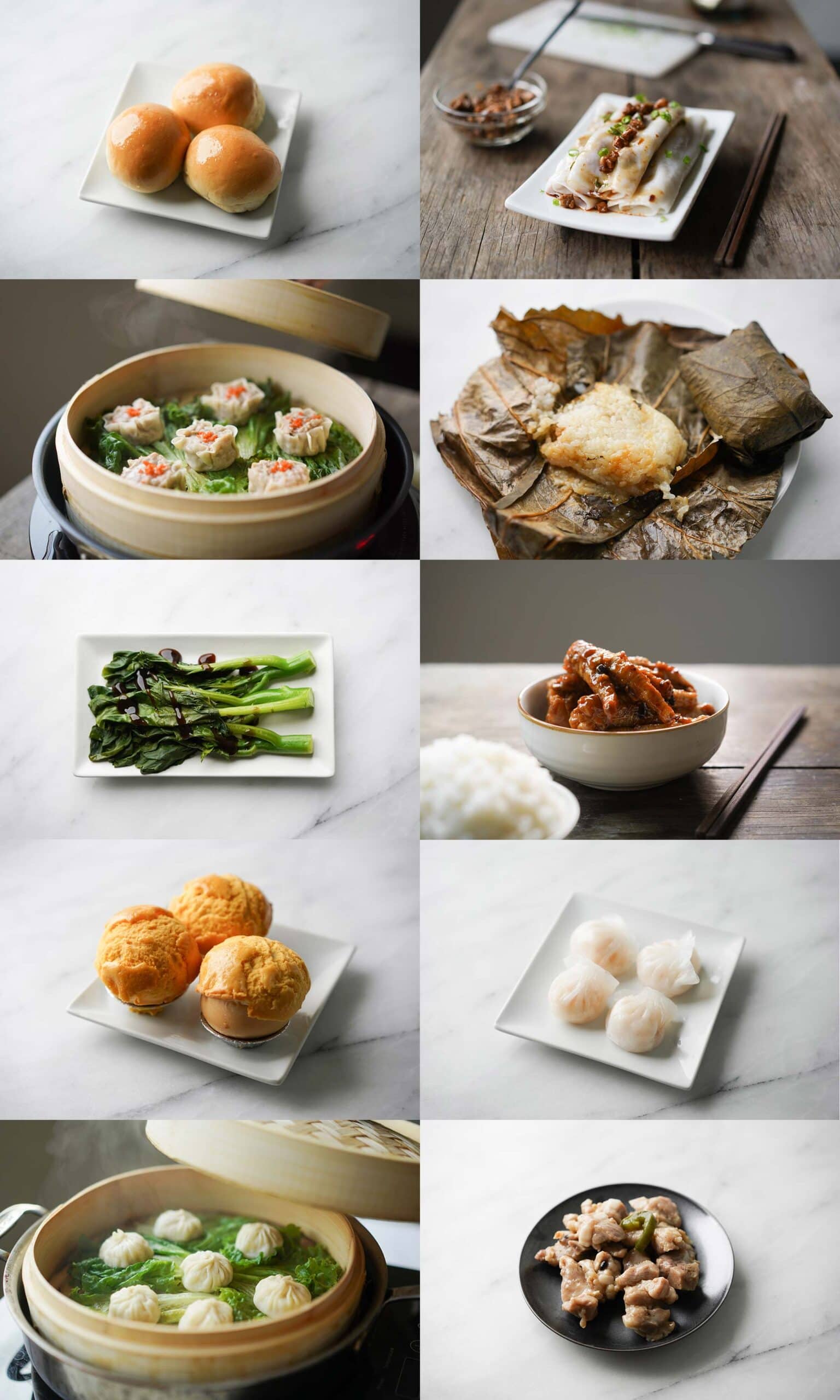
Finally, I got it working! I could print out the menu, search for dim sum items by name, and even add new items to the menu. It wasn’t perfect, but it was a fun little project.
What I learned? Well, besides refreshing my Python skills, I realized that even simple tasks like organizing data can be a fun coding challenge. And that proper data cleaning and structuring from the start can save you a lot of headache later on.